Integrating Amazon Cognito Authentication in a React App
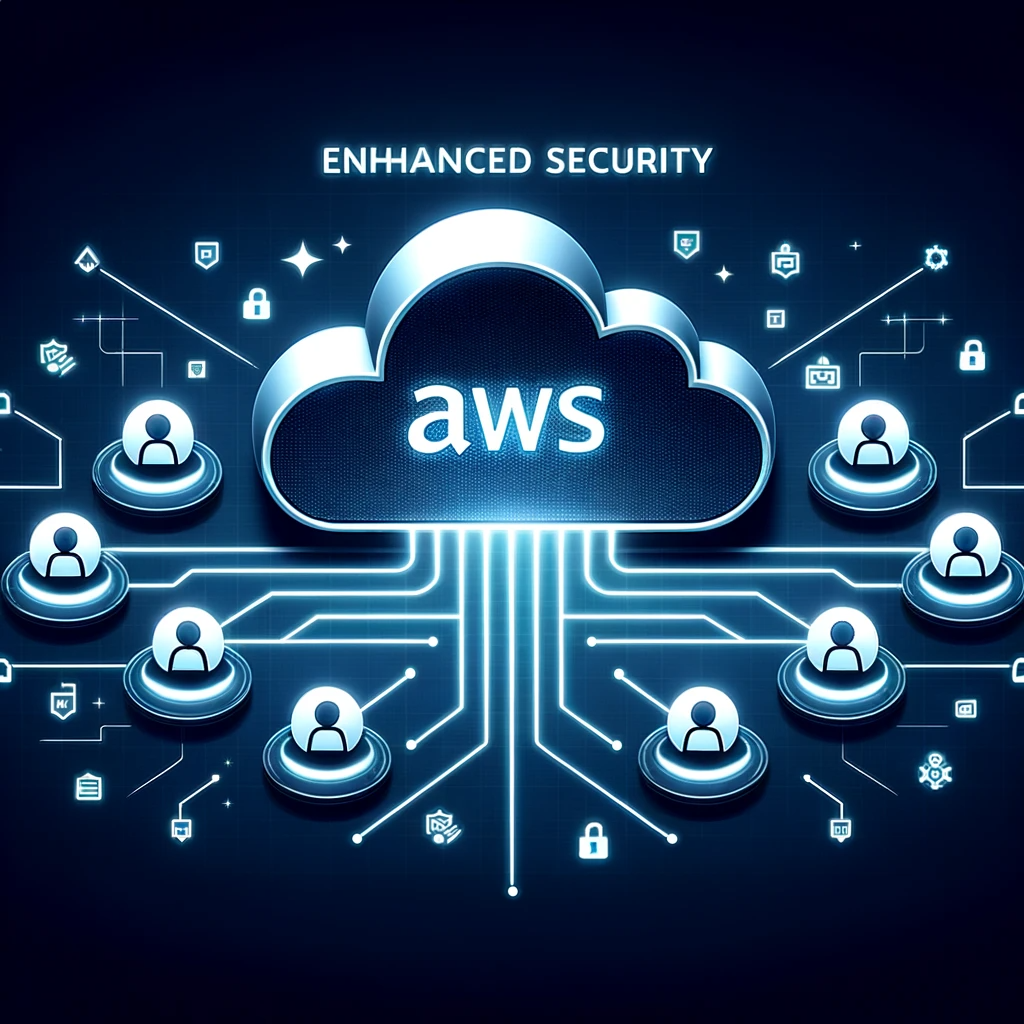
Step-by-step guide on integrating Amazon Cognito authentication in a React app. The guide details the setup from installing Amplify to deploying the app to S3, providing a secure React app leveraging AWS services.
Step-by-step guide on integrating Amazon Cognito authentication in a React app. Follow through the detailed setup from installing Amplify to deploying your app to S3. Build a secure React app leveraging AWS services, complete with code snippets for seamless implementation.
Used resources #
- A youtube video
- it was demonstrated with v1 I was able to up gade it to v2
Setup guide #
Install Amplify #
npm install -g @aws-amplify/cli
install react #
npm install react react-dom --save
create react app #
npx create-react-app name-amplify-react-app
Configure Amplify #
amplify configure
Initiate Amplify setup #
amplify init
During the init we need to answer the following questions:
- App Name
- Environment (dev)
- Default Editor (vscode)
- App type (javascript)
- Javascript framework (react)
- Source Directory Path (src)
- Build command: npm run-script build
- Start command: npm run-script start
- Build provider (cloudformation)
- AWS profile (any of the above, created in the configure phase)
The folder amplify
is created and a file /src/aws-exports.js.
add authentication to the back end #
amplify add auth
I configured it with the following settings:
- setup default configuration
- Sign in usning E-Mail
- No need to setup advanced settings
push the backend to the cloud #
amplify push
- select the proposed name and continue
insert the code for cognito #
Open the file /src/App.js
We need to import a couple of things:
- Import amplify items
- replace the code for our application
- replace the default export wit the export that includes authentication
Import amplify items #
Replace the import codeblock at the top of the file:
import { Amplify } from 'aws-amplify';
import { withAuthenticator } from '@aws-amplify/ui-react';
import '@aws-amplify/ui-react/styles.css';
import awsExports from './aws-exports';
import React from 'react';
import './App.css'
Amplify.configure(awsExports);
Replace the code for our application #
Remove all content between <header classname=App-header>
and </header>
elements.
and replace it by the following:
<div>
<h1>Hello {user.username}{user.emailaddress}</h1>
<p>this is just a test to see if it works</p>
<p>Is it automaticly deployed?</p>
<button class="amplify-button amplify-field-group__control amplify-button--primary" onClick={signOut}>Sign out</button>
</div>
replace the default export #
export default withAuthenticator(App);
install final npm packages #
npm install --save aws-amplify @aws-amplify/ui-react
test react app locally #
npm start
Your application should start localy #
deploy the code to the S3-bucket #
cd .\amplify-react-app
npm run build
aws s3 sync build/ s3://react.amazoninstructor.info --delete